What would any game be without a little randomness? Here is a shuffle bag with the Fisher-Yates shuffle algorithm.
We are going to imagine this is a test for a trading game in which players grab resources from a pool of resources i.e the shuffle bag. Some resources are rarer than others and appear less. Each resource also has a value. Also, when a resource is drawn, we want to remove it from the random picking of the remaining resources. When our shuffle bag is empty, we re-fill it and go again.
There will be three classes used for the scene: a shuffler, a manager, and a displayer. A shuffle bag class contains functions for shuffling a list and making changes to it, like inserting or removing elements. A manager creates and fills shuffle bags. Lastly, a display class handles the user interface.
Lets start with the Shuffle Bag.
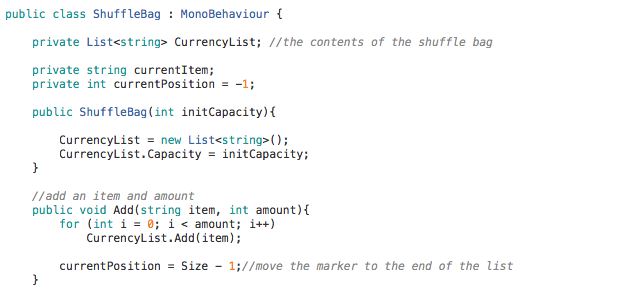
The shuffle bag will have a constructor, so we can set how many elements it can hold when we create an instance of it with our manager class. Right now, there is just a function for Add which allows a type of item to be added a specified amount of times. All of those items are added to our CurrencyList, and we move a place holder to the end of the list.
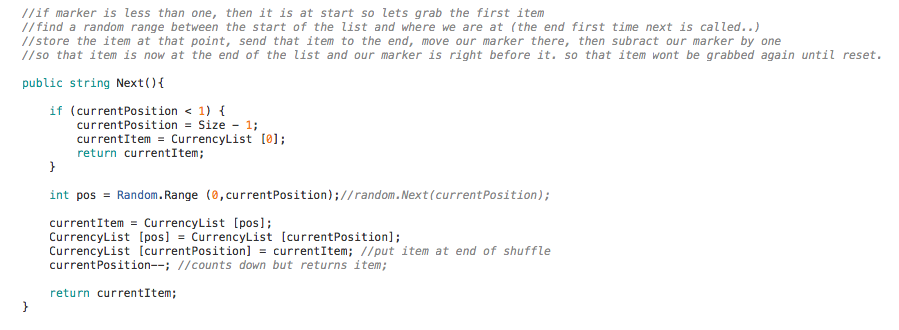
The Next() function will randomly grab an item from our bag and shuffle the contents in the bag. This is the main part of the shuffle algorithm. If our marker is less than one, we are indexed at the first element of the list so now we know our location to grab the first item.
Then, it finds a random range between the start of the list and where we are at. It stores the item at that point, then sends that item to the end, then moves our marker there, then subtracts our marker by one so that item is now at the end of the list, and our marker is right before it. That item will not be grabbed again until a reset.
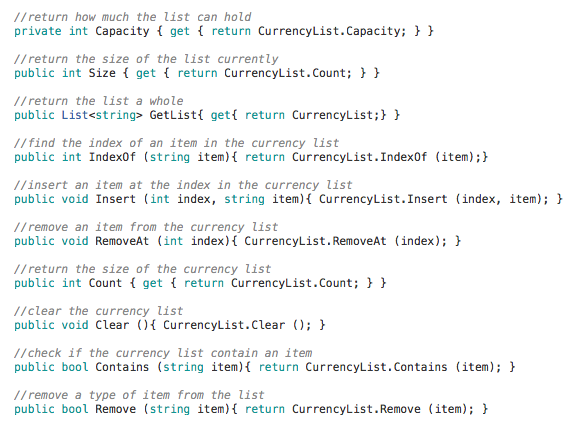
For more control over our shuffle bag, we can add many different helper functions to interact with the list of contents.
A manager class will take care of making the shuffle bags and filling them with contents as you see below.
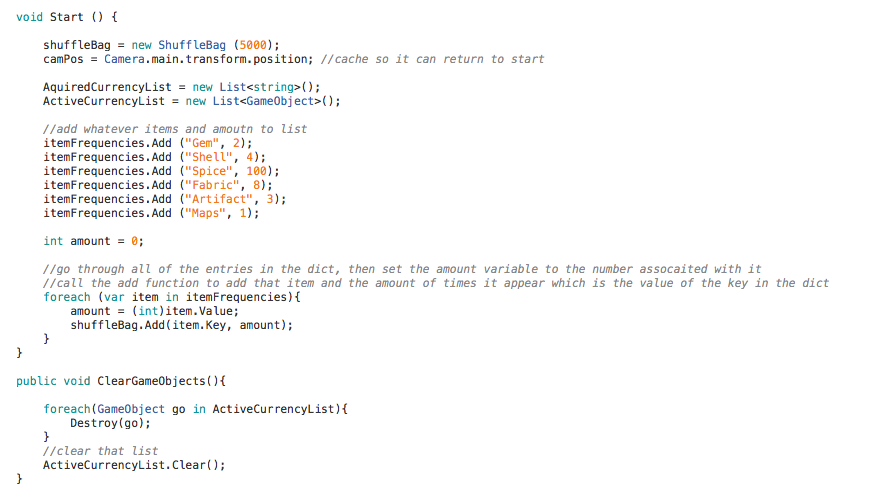
We start with creating a new instance of ShuffleBag then set up our list to keep track of items. ItemFrequencies will allow us to specify the type of item and the amount of times it will be added to the shuffle bag list. ItemFrequencies is a dictionary of type string, double. We start with creating a dictionary of type string, double. The double variable will be the number of how many times that item is added to the bag. Then, we loop through all of the items and pass them and their amount to the add function. The add function then loops through the value of the item name and adds that item corresponding to its value, adding it to an overall list called the CurrencyList. As the size of the list increases so does our marker until we are at the end.
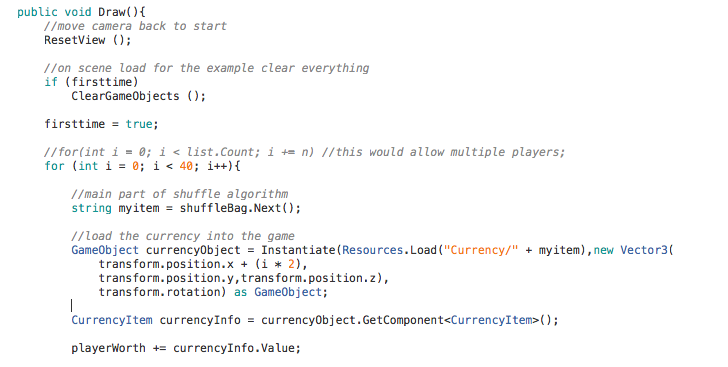
To draw an object from the list, we are going to iterate over a for loop however many times and create objects from the shuffle bag. Each item in the shuffle bag corresponds to a prefab in the resource folder, so that the path name plus the item name is used to instantiate the object. Then, we grab information from that item such as how much it is worth.
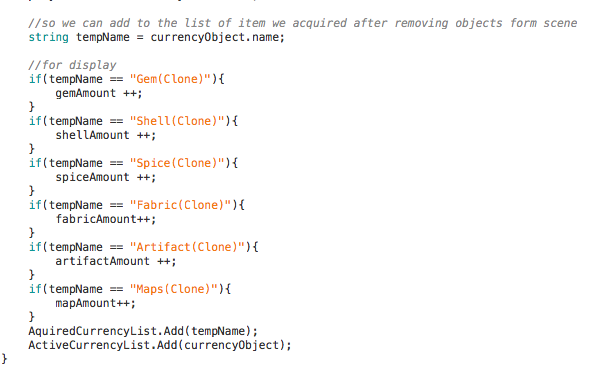
Now that we made a Game Objects to represent the dictionary string, we need to add that to a currency list for our score and a currency list for the visual Game Object. As the for loop runs, if the item name matches one of the current items,we increment that amount.
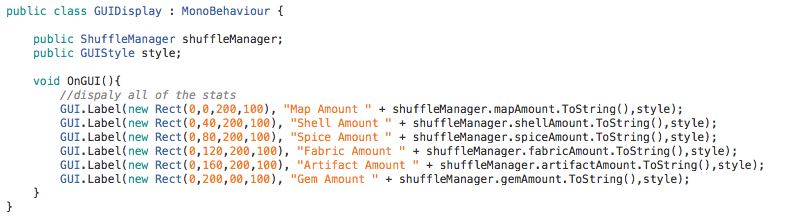
In a separate display class,we get a reference to the shuffle manager and set the score.